Bubble Battle
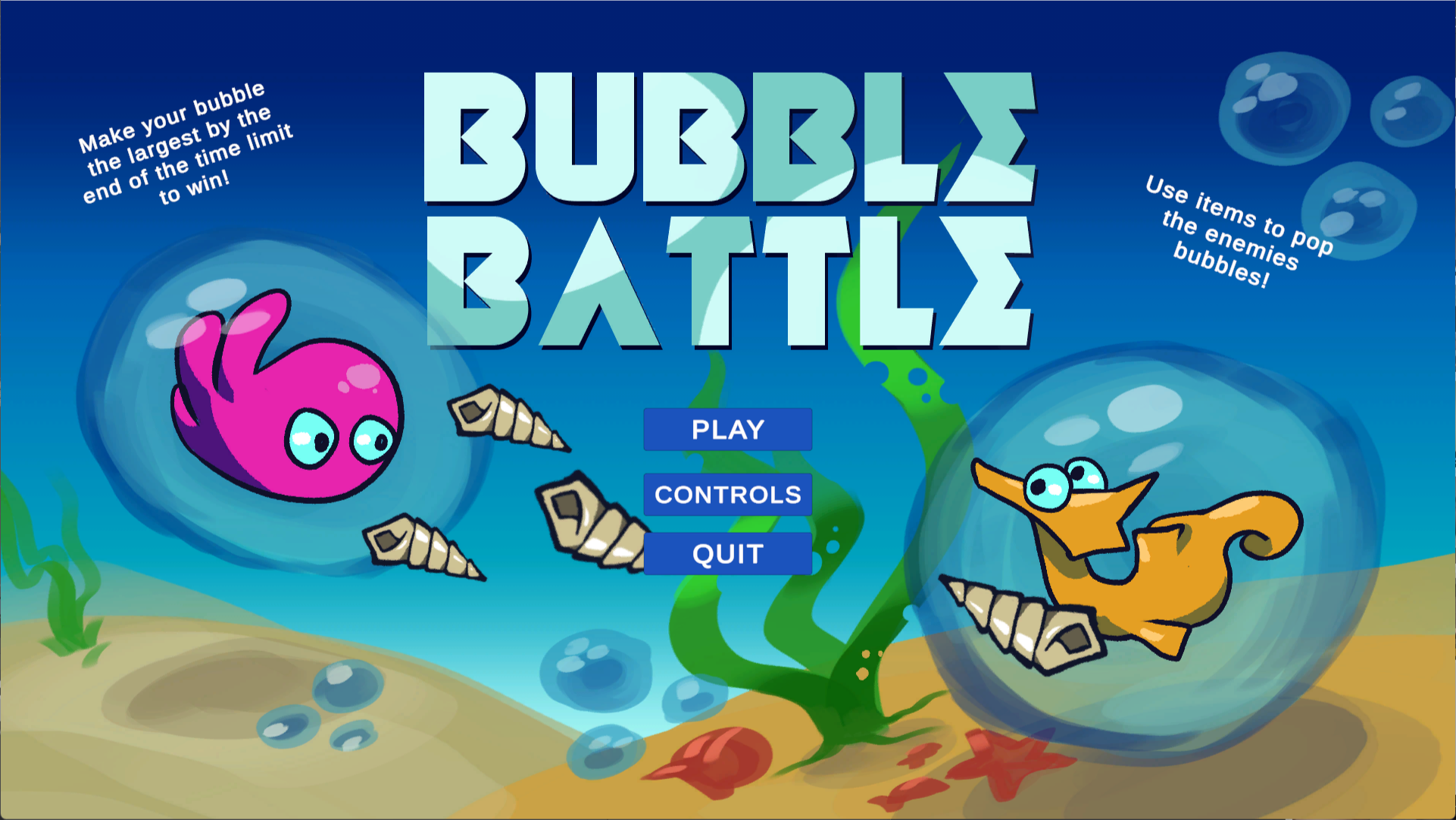
Download the Mac game and source code here!
My first Global Game Jam went without a hitch! Completing a game within 48 hours is a feat on its own. What if it's with people you just met in the room? Oh. Also, what if the game should fit a theme that's revealed minutes before you find your team? We did all of that!
The internet, and even the official GGJ website, has many articles about becoming a jammer, so I won't go into that. Instead, I'm briefly offering my perspective as a seasoned engineer on my first time as a jammer.
The GGJ
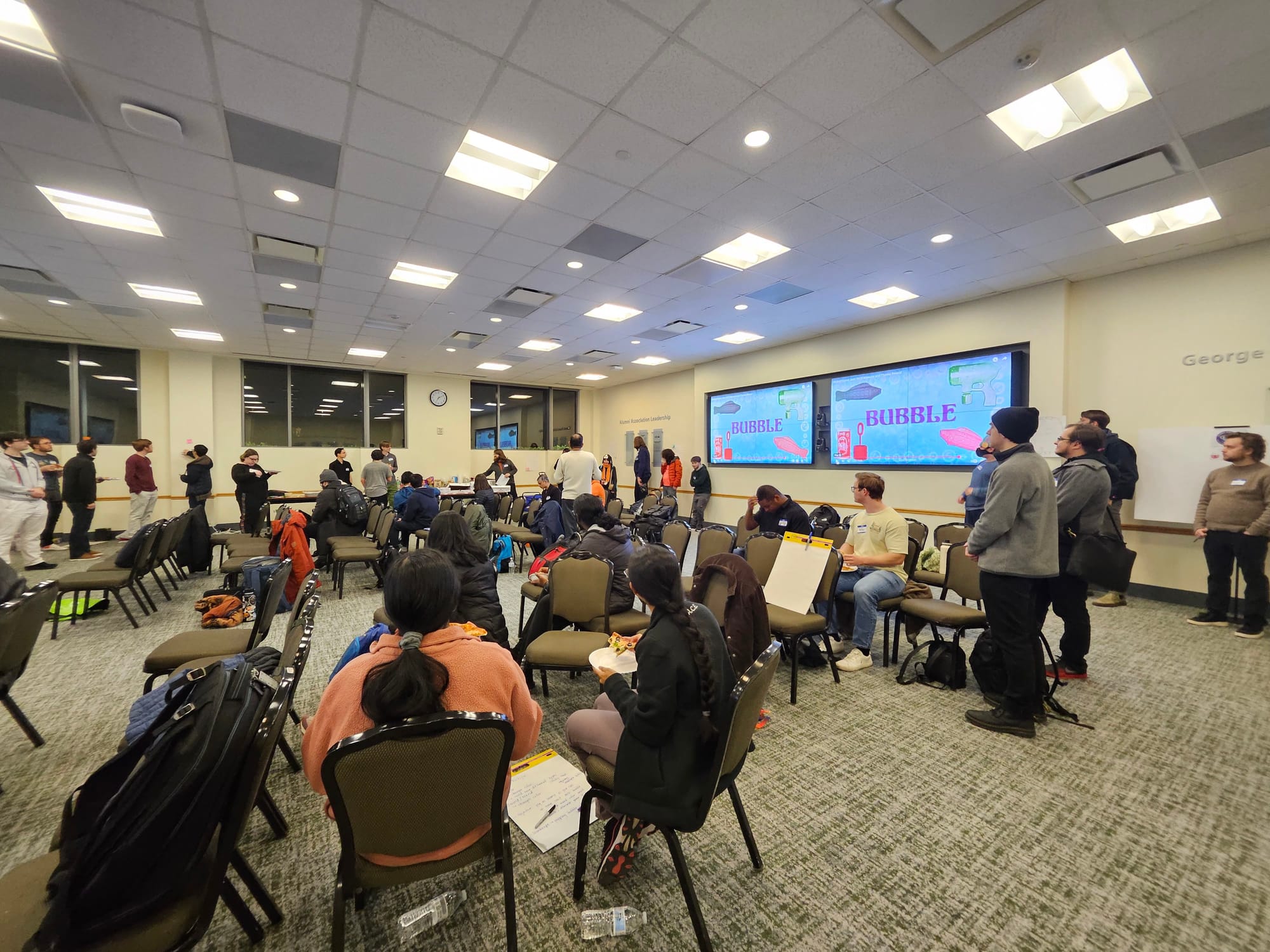
George Mason University gave us a grand start to the festivities with this theme reveal video! At this point in the night, I was concerned about finding a team to help me make a "Bubble" themed game. Is it standard practice at game jams to put color-coded stickers, i.e. red for programmers, on your name tag? That helped me spot artists and programmers. A complete surprise to me was that the people who I sat next to all became my teammates after the theme reveal. We exchanged game ideas and it just worked out that way.
The first night went by faster than I imagined. Most of us simply got the tools needed for the rest of the game jam and went home. The next day was an exhilarating whirlwind of collaboration, progress, and coffee trips. The last day easily won as the most stressful day of the event, but it was worth it. We had a finished, polished game! The work that could be done after submission was correctly delegated into the "Wish List" column.
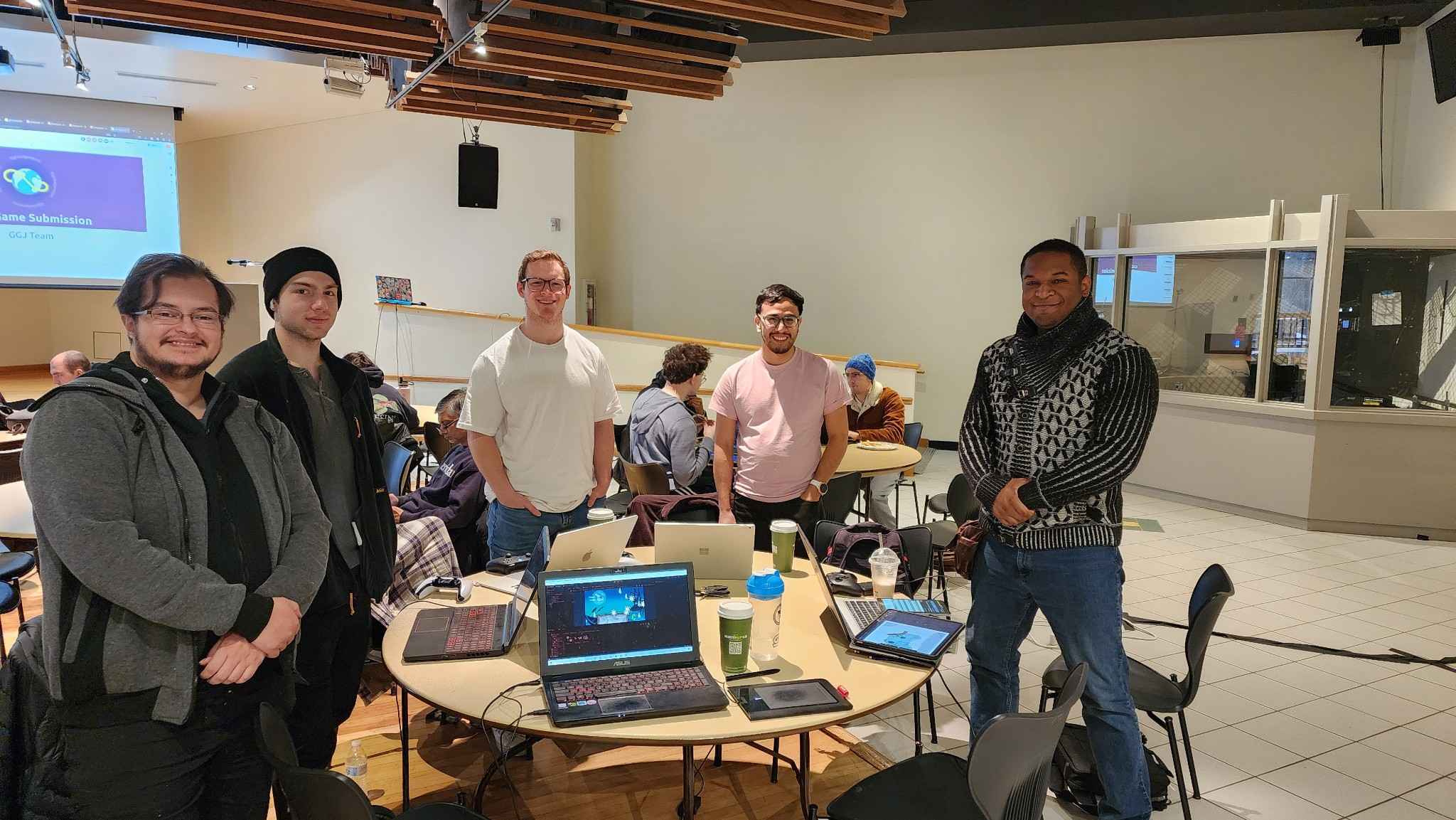
On AI
Something I quickly noticed during development was how much my teammates got done using AI-generated code. Now, I have been critical of AI in the past, mostly due to how AI "creativity" is trained on images it finds on the internet, but I've been lax on generated code because it's easier for AI to show attribution for text. I have used AI for some professional work but it's safe to say that I'm a late adopter.
That being said, AI and game jams go together like peanut butter and jelly. It's the right kind of code for a 2-day project with a presentation to make. We used AI to allow us to make a larger game than what we might have attempted without it. Yes, some game jams don't allow it, but the GGJ does. My takeaway is to learn to freely use AI to help scaffold ideas. For game jams the scaffolding is the final product, so let the AI rip.
Post-GGJ: "Fixing" the bomb
In Bubble Battle's gameplay, a known issue with the bomb happens when you fire it while facing towards the right. The bomb should explode but it will look like it implodes. Huh? While this looks cool it wasn't what the team had expected.
Projectiles swirl differently based on the heading.
I tackled this problem by simplifying it to gain clarity. I like to think of this as if I'm running a binary search for the issue. I find code that, when removed, doesn't affect the problem I'm seeing and by repeating that process eventually all that's left is problematic code.
In this issue, I noticed that the bomb's projectiles were inheriting unnecessary transform details from the parent like scale and rotation. There has to be a nice way to say this, but I split the parent-child relationship. After changing that the explosions stayed consistent no matter which way the player faces.
GameObject projectile = new GameObject("Projectile");
// From this
projectile.transform.SetParent(transform);
projectile.transform.localPosition = new Vector3(xAngle * _radius, yAngle * _radius, 0);
// To this
projectile.transform.position = new Vector3(
xAngle * _radius + transform.position.x,
yAngle * _radius + transform.position.y,
0
);
Projectiles without a parent.
The ideal version
I could stop here but I wanted to know how the twisting effect worked. During the game jam I assumed it was something in Unity's physics engine affecting the velocity. Here's the effect:
Bad angle
Aha, now I see that the projectiles start at an angle so that they move along the edges of the bomb. Slowing down the effect made that easy to spot. Here's the code that sets those angles:
/*
* Rotate the projectile using Atan2 to get the angle between the
* projectile and the center of the circle
*/
float angleOffset = (float) Math.PI/4;
float angleInDegrees = (Mathf.Atan2(yAngle, xAngle) + angleOffset) * Mathf.Rad2Deg;
projectile.transform.rotation = Quaternion.Euler(0, 0, angleInDegrees);
This was so close! At the game jam I tried a few different angleOffset
angles and stopped at Math.PI/4
because it created that swirl effect. The angle that I intended to use was 90°, or Math.PI/2
. Except that didn't look correct either:
Not quite there yet
If I flip this by 180°, i.e. set angleOffset
to -90°, -Math.PI/2
, things look better.
That's it!
Now let's see how it looks in the game with 10 projectiles.
Future game jams
I enjoyed the passion that everyone brought to this event. My first game jam couldn't have gone better. I went into this blind, I didn't prepare, so I know I'll have goals for the next time I attend a jam. For now, I'm re-learning Unreal Engine and hope to try that out for a jam in the future. Until next time!
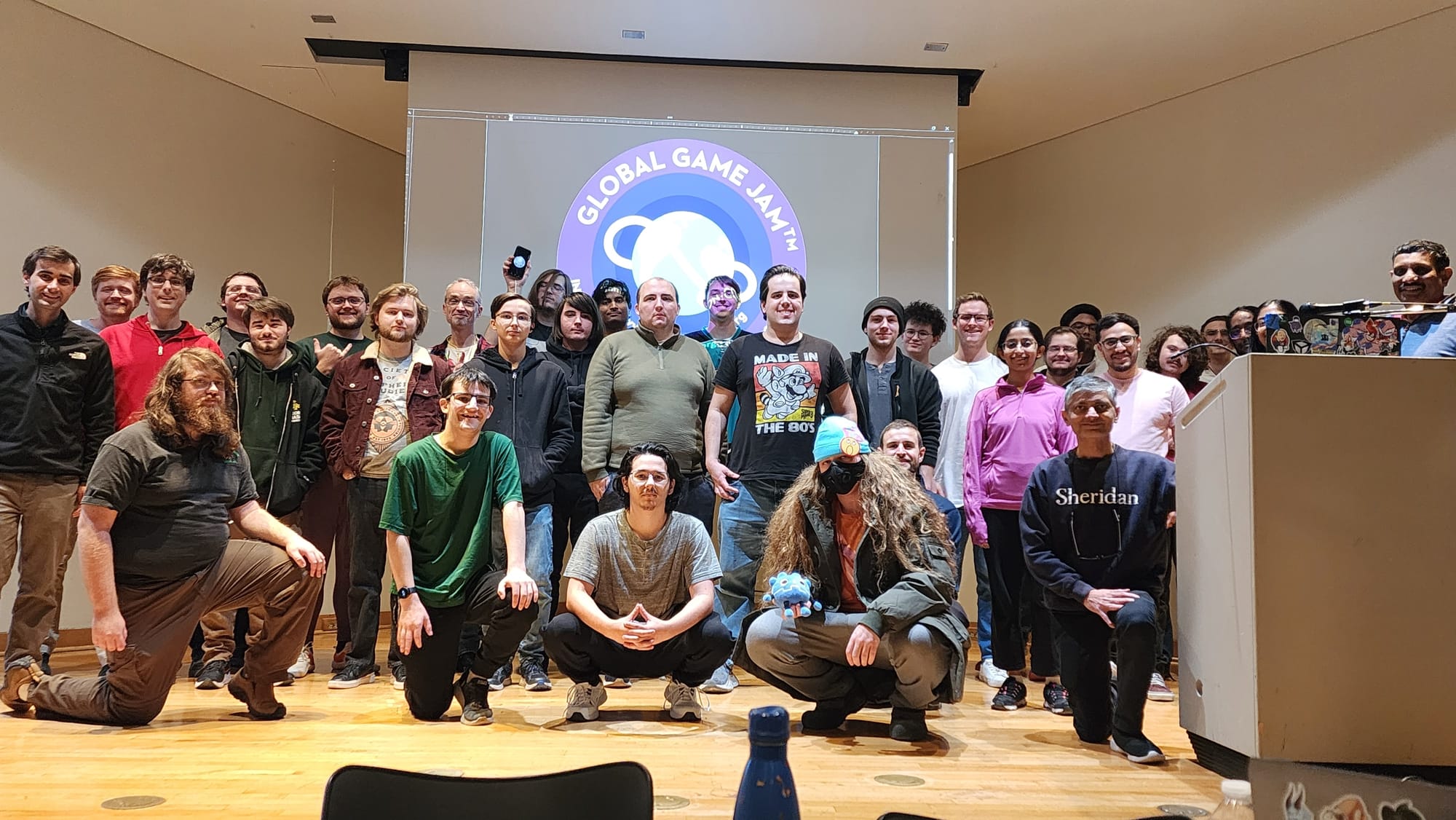